Spring 입문 / 2. Spring Web 개발 기초
by rlaehddnd04221. 정적 컨텐츠
- Spring boot는 정적 컨텐츠 기능 제공
정적 콘텐츠 : html을 그대로 내려주는 방식
그림과 같이 톰켓 서버에서 요청받아, 우선적으로 Controller에서 hello-static.html을 찾아보고 hello-static 관련 컨트롤러가 없으면 그대로 리턴한다.
2. MVC와 템플릿 엔진
MVC, Template : 변형해서 내려주는 방식
- MVC ( Model + View + Controller )
그림과 같이 내장 톰켓 서버에서 요청받은 페이지를 스프링 컨테이너의 Controller에 매핑된 name이 있으면 해당 name의 model을 통해 처리한 후 return 타입이 문자열(viewName)이면 viewResolver를 통해 viewName.html을 처리하고 HTML 변환 후 리턴
Controller
@Controller
public class HelloController {
@GetMapping("hello-mvc")
public String helloMvc(@RequestParam("name") String name, Model model) {
model.addAttribute("name", name);
return "hello-template";
}
}
View
resources/templates/hello-templates.html
<html xmlns:th="http://www.thymeleaf.org">
<body>
<p th:text="'hello ' + ${name}">hello! empty</p>
</body>
</html>
3. API
- @ResponseBody 문자 반환
@ResponseBody를 사용하면 ViewResolver를 사용하지 않음 ( No return html )
대신에 http의 body(html body tag X)에 문자 내용을 직접 반환 ( return string )
@Controller
public class HelloController {
@GetMapping("hello-string")
@ResponseBody
public String helloString(@RequestParam("name") String name) {
return "hello " + name;
}
}
실행
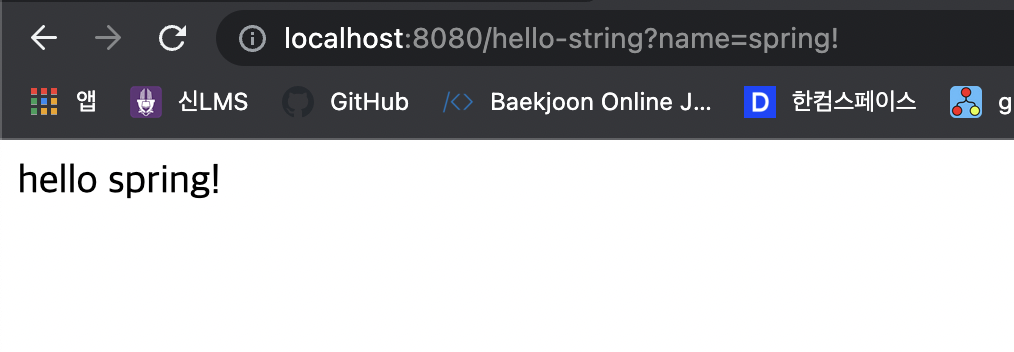
@ResponseBody
public String helloString(@RequestParam("name") String name)
{
return "hello " + name;
}
@RequestParam("name") 을 통해서 파라미터로 name을 받아 ( @ResponseBody 사용했기 때문에 ) http의 body로 리턴
- @ResponseBody로 객체 반환
@ResponseBody를 사용하고 리턴타입을 객체로 설정하면 객체가 json으로 변환됨
@Controller
public class NewController2 {
@GetMapping("hello-api")
@ResponseBody
public Hello helloApi(@RequestParam("name") String name)
{
Hello hello = new Hello();
hello.setName(name);
return hello;
}
static class Hello
{
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
}
실행
정리
@ResponseBody를 사용 -> HttpMessageConverter가 동작
-> http의 body에 문자 내용을 직접 반환해서 보여줌
-> viewResolver(뷰 리졸버) 사용하지 않고, HttpMessageConverter가 동작
-> 기본문자처리 : StringHttpMessageConverter
-> 기본객체처리 : MappingJackson2HttpMessageConverter
참고: 클라이언트의 HTTP Accept 해더와 서버의 컨트롤러 반환 타입 정보 둘을 조합해서 HttpMessageConverter 가 선택된다.
<참고 자료>
[무료] 스프링 입문 - 코드로 배우는 스프링 부트, 웹 MVC, DB 접근 기술 - 인프런 | 강의
스프링 입문자가 예제를 만들어가면서 스프링 웹 애플리케이션 개발 전반을 빠르게 학습할 수 있습니다., - 강의 소개 | 인프런...
www.inflearn.com
'📕 Backend' 카테고리의 다른 글
Spring 입문 / 6. DB 접근 기술 (0) | 2023.01.19 |
---|---|
Spring 입문 / 5. 회원 관리 예제 - 웹 MVC 개발 (1) | 2023.01.17 |
Spring 입문 / 4. 스프링 빈과 의존관계 (0) | 2023.01.17 |
Spring 입문 / 3. 회원 관리 예제 - 백엔드 개발 (0) | 2023.01.16 |
Spring 입문 / 1. 프로젝트 환경설정 (0) | 2023.01.12 |
블로그의 정보
Study Repository
rlaehddnd0422